Big O Notation is a mathematical expression that describes how much time an algorithm takes to run according to the size of it's inputs; mostly concerned about the worst case scenario.
Types:
1- Constant Time O(1):
On this order, regardless of the number of items, the iterations(time) are constant.
Example:
const getFirstItem = items =>
items[0];
getFirstITem([1, 2, 3, 4]); // 1 (one iteration)
getFirstItem(['b', 'd', 'g']); // 'b' (one iteration)
2- Linear Time O(n):
On this order, the worst case grows with the number of items.
Example:
Javascript's built in function indexOf, it loops over an array to find the correct index of the passed element. The worst case is looping over the whole array.
[1, 2, 4, 9, 23, 12].indexOf(12);
3- Quadratic Time O(n ^ 2):
For this order, the worst case time is the square of the number of inputs. It grows exponentially according to the number of inputs.
Example:
Using nested loops would be a good example, or using a loop with a built in function that loops over the elements like indexOf.
for(let i=0; i<arr.length; i++){
if(arr.indexOf('j')){
//do something
}
}
4- Logarithmic Time O(log n):
These are the best for searching and sorting algorithms, they usually are most efficient when dealing with large collections. There's no need to look through the components one by one; but splitting the data to parts and discard some of them at every iteration.
Example:
Great examples on this includes merge sort, binary search and quick search.
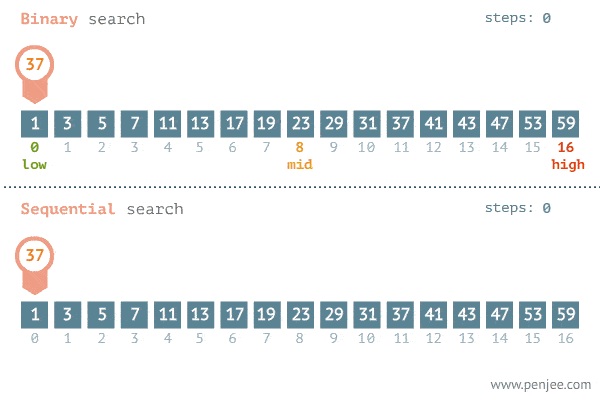
These are the most common of them, and as a conclusion I leave you with a chart that summarizes what we have talked about thus far.

Types:
1- Constant Time O(1):
On this order, regardless of the number of items, the iterations(time) are constant.
Example:
const getFirstItem = items =>
items[0];
getFirstITem([1, 2, 3, 4]); // 1 (one iteration)
getFirstItem(['b', 'd', 'g']); // 'b' (one iteration)
2- Linear Time O(n):
On this order, the worst case grows with the number of items.
Example:
Javascript's built in function indexOf, it loops over an array to find the correct index of the passed element. The worst case is looping over the whole array.
[1, 2, 4, 9, 23, 12].indexOf(12);
3- Quadratic Time O(n ^ 2):
For this order, the worst case time is the square of the number of inputs. It grows exponentially according to the number of inputs.
Example:
Using nested loops would be a good example, or using a loop with a built in function that loops over the elements like indexOf.
for(let i=0; i<arr.length; i++){
if(arr.indexOf('j')){
//do something
}
}
4- Logarithmic Time O(log n):
These are the best for searching and sorting algorithms, they usually are most efficient when dealing with large collections. There's no need to look through the components one by one; but splitting the data to parts and discard some of them at every iteration.
Example:
Great examples on this includes merge sort, binary search and quick search.
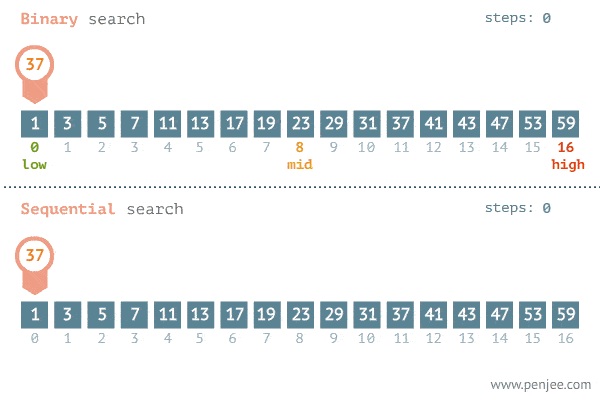
These are the most common of them, and as a conclusion I leave you with a chart that summarizes what we have talked about thus far.

Source: http://bigocheatsheet.com/
Comments
Post a Comment